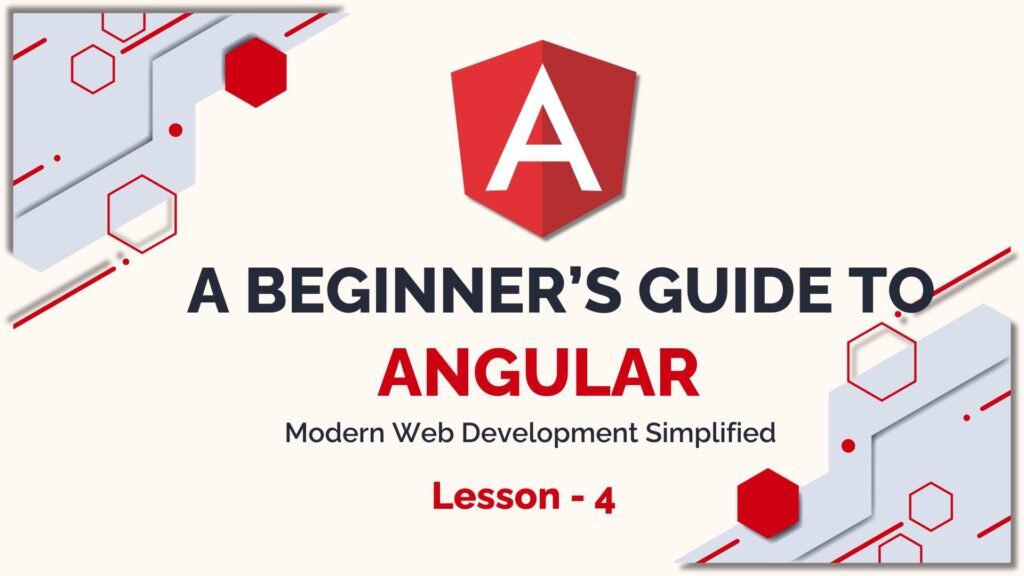
8. Angular Routing and Navigation
Routing in Angular is a key feature that allows you to navigate between different views or pages in your application. In this lesson, we will cover how to set up routing, create routes, and navigate between them.
1. Introduction to Angular Routing
Angular provides a RouterModule that enables the configuration of routes within your application. This module helps in:
- Navigating between views.
- Passing data between different components or views.
- Controlling how the user moves through your app (e.g., guarding routes for authentication).
2. Setting Up Routing
To use routing in an Angular application, you first need to set up the RouterModule
in your app. This can be done by importing it into your app module and defining routes.
Steps to Set Up Routing:
- Import
RouterModule
andRoutes
into theapp.module.ts
file. - Define the routes for different components in your application.
- Apply
RouterModule.forRoot()
in theimports
section of yourAppModule
.
Example:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { RouterModule, Routes } from '@angular/router';
import { AppComponent } from './app.component';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
// Define routes
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
@NgModule({
declarations: [
AppComponent,
HomeComponent,
AboutComponent
],
imports: [
BrowserModule,
RouterModule.forRoot(routes) // Set up the routes
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
3. Creating Routes
- Path: Each route is defined with a path that determines the URL structure.
- Component: Each path is associated with a component that Angular will display when the route is visited.
For example:
'about'
: The URL/about
will display theAboutComponent
.'home'
: The URL/home
will display theHomeComponent
.
4. Using the RouterLink Directive
To navigate between different routes, Angular provides the RouterLink
directive. This directive allows you to link to specific routes within your template.
Example:
<nav>
<a routerLink="/">Home</a>
<a routerLink="/about">About</a>
</nav>
<!-- Routed components will be displayed here -->
<router-outlet></router-outlet>
The <router-outlet>
directive is used to define the space where routed components will be displayed.
5. Navigating Programmatically
In addition to the RouterLink
directive, you can navigate programmatically in your component’s TypeScript file by using the Router
class.
Example:
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-home',
template: `<button (click)="goToAbout()">Go to About</button>`
})
export class HomeComponent {
constructor(private router: Router) {}
goToAbout() {
this.router.navigate(['/about']);
}
}
6. Route Parameters
Angular routes can also accept parameters, allowing you to pass dynamic data through the URL. This is useful for details pages or editing specific items in a list.
Example of Defining Route with a Parameter:
const routes: Routes = [
{ path: 'product/:id', component: ProductComponent }
];
Example of Accessing Route Parameters: In your component, you can access the route parameters using ActivatedRoute
.
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-product',
template: `<p>Product ID: {{ id }}</p>`
})
export class ProductComponent implements OnInit {
id: string | null = '';
constructor(private route: ActivatedRoute) {}
ngOnInit() {
this.id = this.route.snapshot.paramMap.get('id');
}
}
7. Route Guards: Protecting Routes
Route guards in Angular are used to protect certain routes from being accessed without meeting specific conditions, like authentication. You can implement guards to control access to specific parts of your application.
Angular offers several types of guards:
- CanActivate: Controls access to a route.
- CanDeactivate: Handles leaving the route.
- Resolve: Pre-fetches data before navigating to the route.
Example of Creating a Guard:
import { Injectable } from '@angular/core';
import { CanActivate, Router } from '@angular/router';
@Injectable({
providedIn: 'root'
})
export class AuthGuard implements CanActivate {
constructor(private router: Router) {}
canActivate(): boolean {
const isAuthenticated = /* check user authentication logic here */ false;
if (!isAuthenticated) {
this.router.navigate(['/login']);
return false;
}
return true;
}
}
To apply a guard, add it to the route definition:
{ path: 'dashboard', component: DashboardComponent, canActivate: [AuthGuard] }
This concludes Lesson 4 on Angular Routing and Navigation. This lesson introduces how to implement navigation between components, set up route guards, and handle dynamic parameters, providing you with the ability to create a fully functional multi-page Angular app.