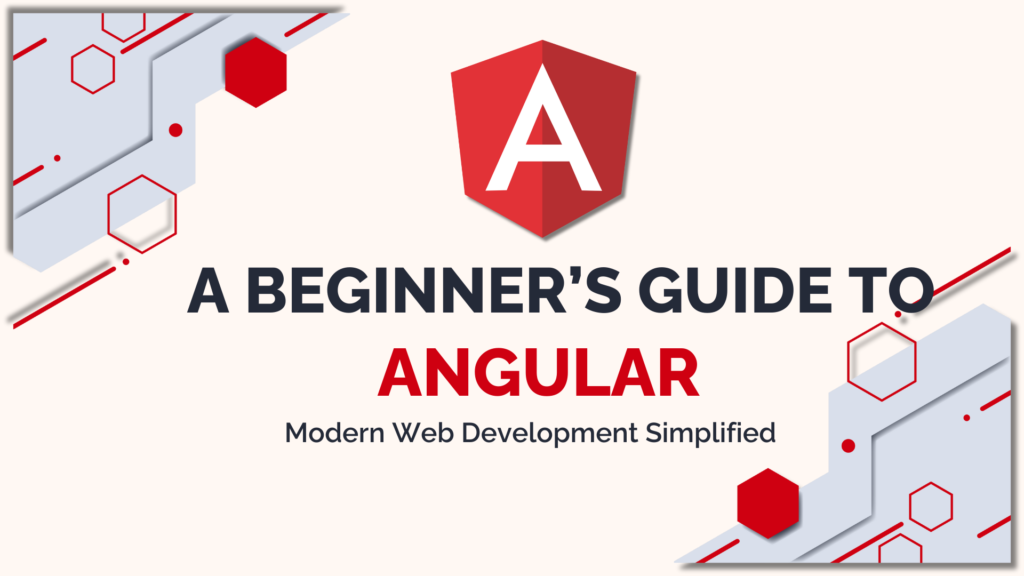
1. Introduction to Angular
Overview of Angular and Its Significance
Angular is a comprehensive, open-source framework developed and maintained by Google, designed specifically for building dynamic single-page web applications (SPAs). It provides a well-structured environment for both small and large-scale applications, simplifying the development process with built-in tools like data binding, dependency injection, and component-based architecture.
One of Angular’s key advantages is its emphasis on clean code and maintainability. By dividing the application into components, Angular helps developers create modular and reusable code. Additionally, the framework is known for its strong tooling support, including a command-line interface (CLI) for creating and managing projects, built-in testing tools, and efficient debugging features.
Why Angular is Popular in Modern Web Development
Angular has become a popular choice for web development due to several reasons:
- Component-Based Architecture: Angular’s component-based structure makes it easy to develop and manage large-scale applications. Each part of the UI is represented as an isolated component, making it easier to maintain and reuse.
- Two-Way Data Binding: One of Angular’s most powerful features is its two-way data binding. This ensures that any change made in the UI is instantly reflected in the model and vice versa, which simplifies handling dynamic data.
- Modular Development: With Angular’s modular approach, developers can break down an application into multiple modules, making development scalable, organized, and easier to test.
- TypeScript Support: Angular is built with TypeScript, which adds static typing to JavaScript. This helps in catching errors during development and improves the overall code quality, making the development process more efficient.
- Built-in Tools for Testing and Debugging: Angular comes with a strong suite of testing tools like Jasmine, Karma, and Protractor, ensuring that developers can easily write and execute unit tests, integration tests, and end-to-end tests.
- Active Community and Ecosystem: Angular has a vast and active community, which means a wealth of resources, libraries, and third-party tools are available. Regular updates from Google ensure that Angular stays relevant and well-supported.
Differences Between AngularJS and Angular (Angular 2+)
Angular has undergone significant changes since its first version, AngularJS, which was released in 2010. The newer versions, often referred to as Angular 2+ or just Angular, are completely different frameworks with various improvements.
Feature | AngularJS (1.x) | Angular (2+) |
---|---|---|
Architecture | MVC (Model-View-Controller) | Component-based architecture |
Language | JavaScript | TypeScript |
Performance | Slower due to digest cycles and dirty checking | Improved performance due to change detection via Zones |
Mobile Support | Limited | Full support for mobile development |
Two-Way Data Binding | Built-in two-way data binding | Two-way data binding with more flexibility |
Routing | In-built basic routing | Powerful, flexible router with lazy loading |
Dependency Injection | Manual injection of dependencies | Built-in, more powerful dependency injection system |
Modularity | Less modular | Modular with NgModules, enhancing reusability and maintainability |
In summary, while AngularJS was revolutionary at the time, Angular 2+ brought significant improvements, including better performance, scalability, and modern development practices. The shift to TypeScript has made it easier to build, maintain, and debug larger applications.
2. Key Concepts of Angular
Components: Building Blocks of Angular Applications
Components are the fundamental building blocks of an Angular application. They control a part of the user interface (UI) and consist of three main parts:
- Template: Defines the HTML view for the component.
- Class (TypeScript): Contains the logic and data for the component.
- Styles: Custom CSS or SCSS to style the component.
Each component is declared in an Angular module and can be reused across different parts of the application. The @Component
decorator is used to define a component, as shown below:
import { Component } from '@angular/core';
@Component({
selector: 'app-hero',
templateUrl: './hero.component.html',
styleUrls: ['./hero.component.css']
})
export class HeroComponent {
title = 'Hero Component';
}
selector
: Defines the HTML tag used to embed the component in a template.templateUrl
: Points to the HTML file for the component.styleUrls
: Points to the CSS file(s) for styling the component.
Modules: Organizing an Angular Application
Angular applications are modular by design, which makes them scalable and organized. Modules are used to group related components, directives, pipes, and services into cohesive blocks of functionality. Each Angular application has at least one module, known as the root module (typically called AppModule
), and additional feature modules can be created as needed.
A module is defined using the @NgModule
decorator, and the following is a typical structure of an Angular module:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { HeroComponent } from './hero/hero.component';
@NgModule({
declarations: [
AppComponent,
HeroComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
declarations
: Specifies the components, directives, and pipes that belong to this module.imports
: Lists other modules that this module depends on.providers
: Declares the services this module makes available to the application.bootstrap
: Specifies the root component that Angular should bootstrap to launch the application.
By using modules, Angular encourages lazy loading—the practice of loading only the necessary modules when they are needed, improving the application’s performance.
Templates: HTML with Angular’s Declarative Syntax
Templates in Angular are an extended version of HTML with additional functionality to bind data and handle user events. Angular templates are embedded within components and define the structure of the view.
Key template features include:
- Interpolation: Used to display dynamic data in the HTML view using double curly braces (
{{ }}
).
<h1>{{ title }}</h1>
Property Binding: Links DOM properties to component data.
<img [src]="imageUrl">
Event Binding: Handles user events (like click, input) using parentheses.
<button (click)="onClick()">Click Me</button>
- Directives: Angular provides structural directives (
*ngIf
,*ngFor
) and attribute directives (ngClass
,ngStyle
) to manipulate the DOM based on the component’s data.
Here’s a simple Angular template example:
<div *ngIf="isVisible">
<h1>{{ title }}</h1>
<button (click)="toggleVisibility()">Toggle</button>
</div>
*ngIf
displays the<div>
only whenisVisible
istrue
.{{ title }}
dynamically binds the value of thetitle
property from the component.(click)
binds the button click event to thetoggleVisibility()
function.
Services and Dependency Injection: Reusable Logic Across Components
Services in Angular are used to write logic that is reusable across multiple components. Common use cases for services include data fetching from APIs, business logic, or managing shared state.
Services are defined as classes and are typically provided to Angular’s Dependency Injection (DI) system, which manages the lifecycle and availability of services. Here’s how you define and use a service in Angular:
- Creating a Service:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class HeroService {
getHeroes() {
return ['Hero1', 'Hero2', 'Hero3'];
}
}
The @Injectable
decorator tells Angular that this class can be injected as a service. The providedIn: 'root'
makes this service available application-wide.
Injecting a Service into a Component: You can inject the service into any component’s constructor to access its methods.
import { Component } from '@angular/core';
import { HeroService } from './hero.service';
@Component({
selector: 'app-hero',
templateUrl: './hero.component.html',
styleUrls: ['./hero.component.css']
})
export class HeroComponent {
heroes: string[];
constructor(private heroService: HeroService) {
this.heroes = this.heroService.getHeroes();
}
}
- In this example, the
HeroService
is injected into theHeroComponent
, and itsgetHeroes()
method is used to fetch a list of heroes. This allows for separation of concerns—the component is responsible for displaying data, while the service handles the logic of fetching it.
Summary
- Components handle specific pieces of the UI and logic.
- Modules group related functionality for easy maintenance and scalability.
- Templates define the HTML structure with powerful binding and event-handling features.
- Services encapsulate reusable logic, and Dependency Injection allows easy sharing of services across the application.
Together, these key concepts make Angular a robust framework for building dynamic, maintainable, and scalable web applications.
3. Setting Up the Development Environment
Prerequisites: Node.js and NPM
Before starting with Angular, it’s essential to have Node.js and NPM (Node Package Manager) installed. Node.js allows you to run JavaScript outside the browser, which is crucial for Angular’s development and build tools.
- Node.js: A JavaScript runtime that allows you to run server-side applications and manage packages.
- NPM: The default package manager for Node.js, used to install libraries and dependencies required by Angular.
Steps to Install Node.js and NPM:
- Visit the Node.js official website.
- Download and install the latest stable version of Node.js (it includes NPM).
- After installation, verify both Node.js and NPM by running the following commands in your terminal or command prompt:
node -v
npm -v
Installing Angular CLI
The Angular CLI (Command Line Interface) is a powerful tool that helps in managing Angular projects. It provides commands to create, build, and serve Angular applications easily, while also offering utilities for generating components, services, and modules.
Steps to Install Angular CLI:
- Once Node.js and NPM are installed, you can install the Angular CLI globally by running the following command:
npm install -g @angular/cli
This installs the Angular CLI globally on your system, making the ng
command available in any directory.
To verify the installation, run:
ng version
- This should display the Angular CLI version along with other details about the environment.
Creating Your First Angular Project
With Angular CLI installed, you can now create a new Angular project using a simple command.
Steps to Create a New Project:
- In the terminal or command prompt, navigate to the directory where you want to create your project, and run the following command:
ng new my-first-angular-app
The CLI will ask a few questions:
- Would you like to add Angular routing? (Yes or No) – Choose based on your needs.
- Which stylesheet format would you like to use? (CSS, SCSS, SASS, LESS) – Choose your preferred stylesheet format.
After answering these questions, the CLI will generate a new Angular project with the default structure, and install all necessary dependencies.
Navigate into the newly created project directory:
cd my-first-angular-app
To start the development server and view your Angular app in the browser, run:
ng serve
- The application will be compiled and served locally at
http://localhost:4200/
. You can open this URL in your browser to see the default Angular welcome page.
Project Structure and File Overview
When you create a new Angular project, it comes with a predefined folder structure. Here’s a quick overview of the most important files and folders:
my-first-angular-app/
├── e2e/ # End-to-end tests folder
├── node_modules/ # Installed dependencies
├── src/ # Source code folder
│ ├── app/ # Contains your application’s components, modules, and services
│ │ ├── app.component.ts # Main component logic (TypeScript)
│ │ ├── app.component.html# Main component view (HTML template)
│ │ ├── app.component.css # Main component styles (CSS)
│ │ └── app.module.ts # Root application module
│ ├── assets/ # Static assets (images, fonts, etc.)
│ ├── environments/ # Configuration for different environments (development, production)
│ ├── index.html # Main HTML file
│ ├── main.ts # Main entry point of the application
│ ├── polyfills.ts # Browser compatibility polyfills
│ ├── styles.css # Global styles for the application
│ └── test.ts # Entry point for unit tests
├── angular.json # Angular CLI configuration file
├── package.json # NPM dependencies and scripts
├── tsconfig.json # TypeScript configuration file
├── tslint.json # Linting rules for TypeScript
└── README.md # Project documentation
src/app/
: This is where most of your application’s code lives. It contains the root module (app.module.ts
), the root component (app.component.ts
), and other components you’ll create.app.module.ts
: The root module that declares which components, services, and other Angular features are part of your application.app.component.ts
: This is the root component of the app, which controls the main layout.app.component.html
: The template (HTML) for the root component, which you can customize to create your app’s UI.angular.json
: Configuration file for Angular CLI. It contains settings related to the build process, including paths, environments, and style options.package.json
: Lists all the dependencies installed in your project and scripts used to build, test, or serve the application.styles.css
: The global stylesheet where you can define CSS rules that apply across the entire app.
Next Steps
After setting up the development environment and creating your first Angular project, you can start exploring components, services, routing, and more. The Angular CLI provides a variety of commands to scaffold new features, test your application, and build it for production.
Conclusion
In this guide, we’ve introduced you to the essentials of Angular and laid the foundation for building dynamic, scalable web applications. We started by exploring what Angular is and why it’s a preferred choice for modern web development. Then, we dove into the key concepts like components, modules, templates, and services—each of which plays a critical role in creating well-structured applications. Finally, we walked through setting up the development environment, installing the Angular CLI, and creating your first Angular project, giving you a hands-on understanding of how to begin your journey with Angular.
In the next lesson, we will continue to explore more advanced topics such as routing, data binding, forms, and HTTP client integration, giving you a deeper understanding of Angular’s powerful capabilities.
Stay tuned, and happy coding!