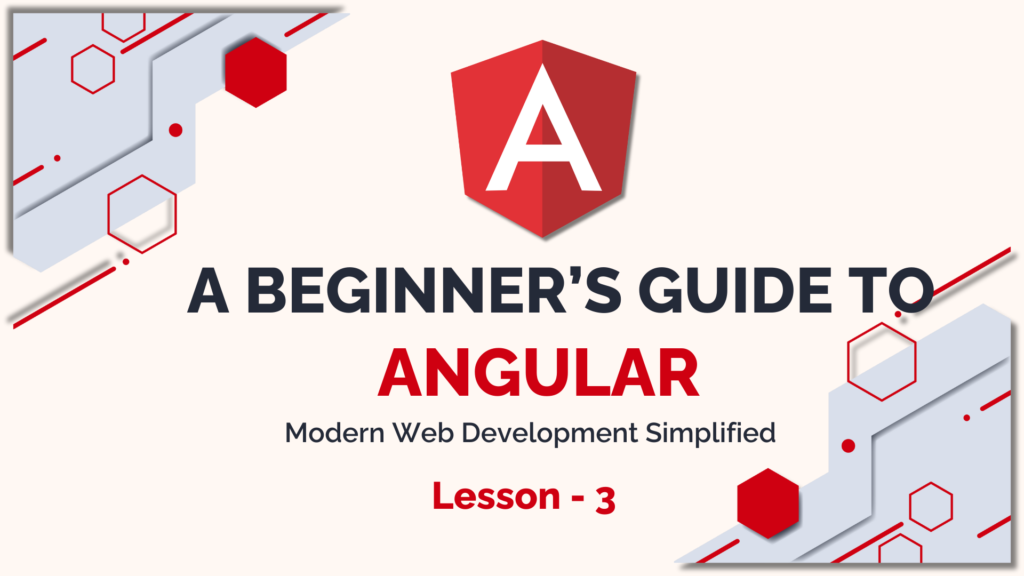
6. Angular Directives
Directives in Angular are instructions added to the DOM, which modify the appearance or behavior of elements. There are two main types of directives: structural and attribute directives.
Structural Directives:
Structural directives change the layout by adding or removing elements from the DOM.
ngIf
Directive:- Displays an element only when a certain condition is true.
- Example:
<div *ngIf="isLoggedIn">Welcome, User!</div>
ngFor
Directive:
- Iterates over a list of data and generates an element for each item.
- Example:
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
ngSwitch
Directive:
- Displays one element from a set of elements based on a matching condition.
- Example:
<div [ngSwitch]="userRole">
<p *ngSwitchCase="'admin'">Admin View</p>
<p *ngSwitchCase="'user'">User View</p>
<p *ngSwitchDefault>Guest View</p>
</div>
Attribute Directives:
Attribute directives change the appearance or behavior of an existing element.
ngClass
Directive:- Dynamically applies or removes a CSS class based on a condition.
- Example:
<div [ngClass]="{ 'active': isActive }">This is a dynamic class example.</div>
ngStyle
Directive:
- Dynamically sets inline styles based on a condition.
- Example:
<p [ngStyle]="{ 'color': isActive ? 'green' : 'red' }">Dynamic Style Example</p>
7. Angular Services and Dependency Injection
Angular services are used to share logic and data across different components. They allow components to be lean and focused on handling user interactions, while the business logic and data retrieval are managed by services.
Creating a Service:
Services are typically used to encapsulate code that is shared across multiple components, such as HTTP requests, data handling, and business logic. You can create a service using the Angular CLI:
ng generate service my-service
This command creates a new service file with the name my-service.service.ts
.
Injecting a Service into a Component:
Services can be injected into components using Angular’s Dependency Injection system.
Example:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root', // This makes the service available throughout the application
})
export class MyService {
getData() {
return 'Service Data';
}
}
// Injecting service in a component
import { MyService } from './my-service.service';
@Component({
selector: 'app-root',
template: `<h1>{{ data }}</h1>`,
})
export class AppComponent {
data: string;
constructor(private myService: MyService) {
this.data = this.myService.getData();
}
}
Why Use Services?
- Code Reusability: Services allow you to keep logic separate and reusable across different components.
- Separation of Concerns: Services help components focus on the user interface, leaving the business logic to the services.
- Dependency Injection: Angular’s DI framework allows services to be injected efficiently, enabling better code management and testing.
In the next lesson, we will continue to explore more advanced topics such as Angular Routing and Navigation giving you a deeper understanding of Angular’s powerful capabilities.