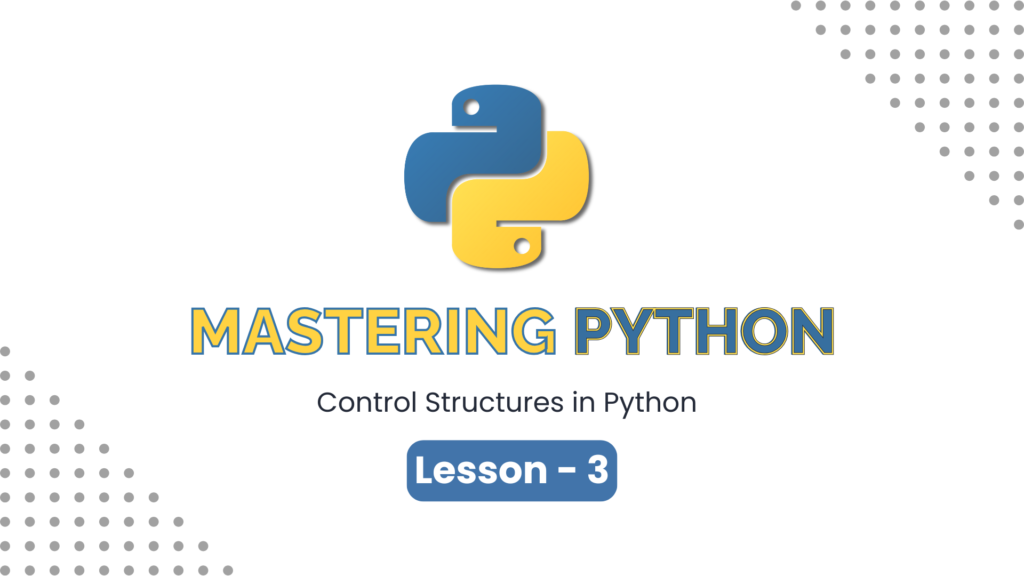
Control structures allow you to control the flow of your Python program by making decisions or repeating tasks. In this lesson, we will cover conditionals, loops, and the concept of indentation in Python.
1. Python Conditionals (if, elif, else)
Conditionals allow your program to make decisions based on specific conditions.
Syntax:
if condition:
# Code block executed if condition is True
elif another_condition:
# Code block executed if the first condition is False and this one is True
else:
# Code block executed if all above conditions are False
Example:
age = 18
if age < 18:
print("You are a minor.")
elif age == 18:
print("Welcome to adulthood!")
else:
print("You are an adult.")
2. Python Loops
Loops are used to execute a block of code repeatedly as long as a condition is met.
a) for Loop
The for
loop is used to iterate over a sequence (like a list, tuple, dictionary, or string).
Syntax:
for variable in sequence:
# Code block to execute
Example:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
b) while Loop
The while
loop continues to execute a block of code as long as the specified condition is True
.
Syntax:
while condition:
# Code block to execute
Example:
count = 0
while count < 5:
print("Count:", count)
count += 1
3. The Concept of Indentation
Python uses indentation to define blocks of code. It replaces the use of curly braces {}
in other programming languages.
Example:
if True:
print("This is part of the if block.") # Indented
print("This is outside the if block.") # Not indented
Incorrect indentation will raise an error:
if True:
print("This will cause an error!") # Missing indentation
4. Nested Loops and Conditionals
You can combine loops and conditionals to create more complex logic.
Example:
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number % 2 == 0:
print(f"{number} is even.")
else:
print(f"{number} is odd.")
5. The break, continue, and pass Statements
These statements are used to alter the normal flow of a loop:
- break: Terminates the loop prematurely.
for num in range(5):
if num == 3:
break
print(num) # Output: 0, 1, 2
continue: Skips the current iteration and moves to the next.
for num in range(5):
if num == 3:
continue
print(num) # Output: 0, 1, 2, 4
pass: Does nothing; acts as a placeholder.
for num in range(5):
if num == 3:
pass # Placeholder
print(num)
6. Using the range() Function
The range()
function generates a sequence of numbers. It is commonly used with for
loops.
Syntax:
range(start, stop, step)
Example:
for i in range(1, 10, 2): # Start from 1, end at 9, step by 2
print(i) # Output: 1, 3, 5, 7, 9
Conclusion
In this lesson, we explored Python’s control structures, including conditionals and loops, which allow you to control the flow of your program. Mastering these concepts will enable you to write dynamic and efficient code. Up next, we’ll dive into functions, where you’ll learn how to encapsulate logic and reuse it across your programs.