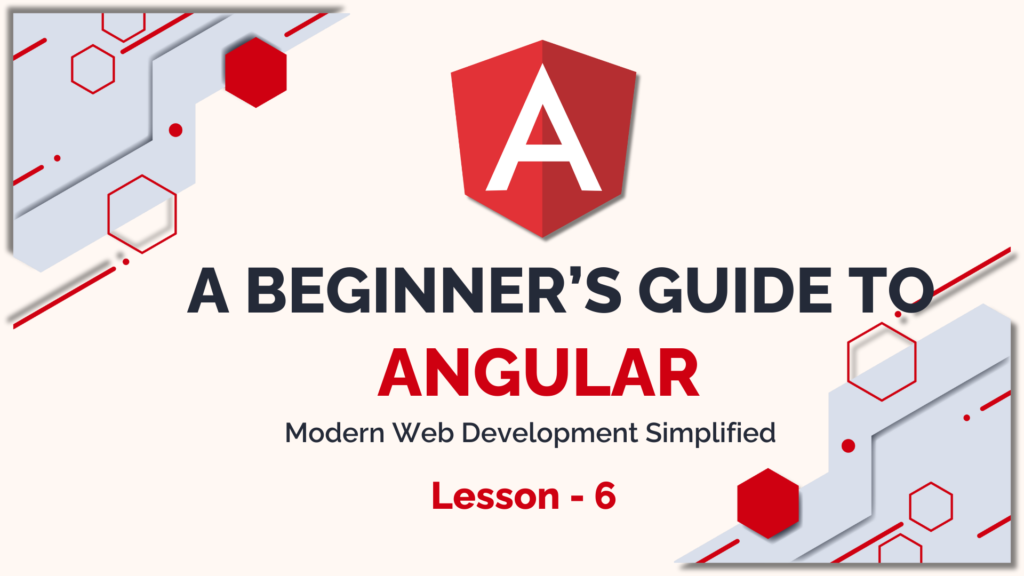
Forms are an integral part of any web application, and Angular provides a powerful way to create and manage forms efficiently. In this lesson, we’ll cover the key concepts of building forms using both template-driven and reactive forms in Angular.
1. Overview of Forms in Angular
Angular offers two approaches to create and manage forms:
- Template-driven forms: Easier to use, great for simple forms.
- Reactive forms: More scalable and flexible, ideal for complex forms and validation logic.
2. Template-driven Forms
Template-driven forms rely heavily on Angular’s two-way data binding to handle user inputs.
Steps to Create a Template-driven Form:
- 1. Import FormsModule in the
app.module.ts
file
import { FormsModule } from '@angular/forms';
@NgModule({
imports: [FormsModule]
})
2. Create a form template using Angular directives like ngModel
for data binding.
Example:
<form #userForm="ngForm" (ngSubmit)="onSubmit(userForm)">
<label for="name">Name:</label>
<input type="text" id="name" name="name" ngModel required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" ngModel required>
<button type="submit" [disabled]="userForm.invalid">Submit</button>
</form>
Handling Form Submission:
export class AppComponent {
onSubmit(form: any) {
console.log('Form Submitted!', form);
}
}
3. Reactive Forms
Reactive forms offer more flexibility and robustness by separating the form logic from the template. This makes it easier to handle complex form scenarios, such as conditional validation and dynamic form controls.
Steps to Create a Reactive Form:
- 1. Import ReactiveFormsModule in
app.module.ts
import { ReactiveFormsModule } from '@angular/forms';
@NgModule({
imports: [ReactiveFormsModule]
})
- Set up form controls in your component’s TypeScript file using
FormGroup
andFormControl
.
Example:
import { Component } from '@angular/core';
import { FormGroup, FormControl, Validators } from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html'
})
export class AppComponent {
userForm = new FormGroup({
name: new FormControl('', Validators.required),
email: new FormControl('', [Validators.required, Validators.email])
});
onSubmit() {
console.log(this.userForm.value);
}
}
Reactive Form Template:
<form [formGroup]="userForm" (ngSubmit)="onSubmit()">
<label for="name">Name:</label>
<input id="name" formControlName="name">
<label for="email">Email:</label>
<input id="email" formControlName="email">
<button type="submit" [disabled]="userForm.invalid">Submit</button>
</form>
4. Form Validation in Angular
Validation is crucial for ensuring that forms receive correct data input. Angular provides built-in validators such as required
, email
, and minlength
, which can be used with both template-driven and reactive forms.
Template-driven Form Validation:
<input type="email" id="email" name="email" ngModel required email>
<div *ngIf="userForm.controls.email.invalid && userForm.controls.email.touched">
Please enter a valid email.
</div>
Reactive Form Validation:
userForm = new FormGroup({
name: new FormControl('', Validators.required),
email: new FormControl('', [Validators.required, Validators.email])
});
5. Dynamic Forms
Angular’s reactive forms also allow you to create forms dynamically by adding or removing form controls at runtime. This is particularly useful when the number of form fields depends on user interaction.
Example:
addControl() {
this.userForm.addControl('phone', new FormControl('', Validators.required));
}
Conclusion
In this lesson, we explored the two main ways of building forms in Angular: template-driven and reactive forms. You now know how to create forms, handle form submissions, implement validation, and even build dynamic forms using Angular’s robust form features.