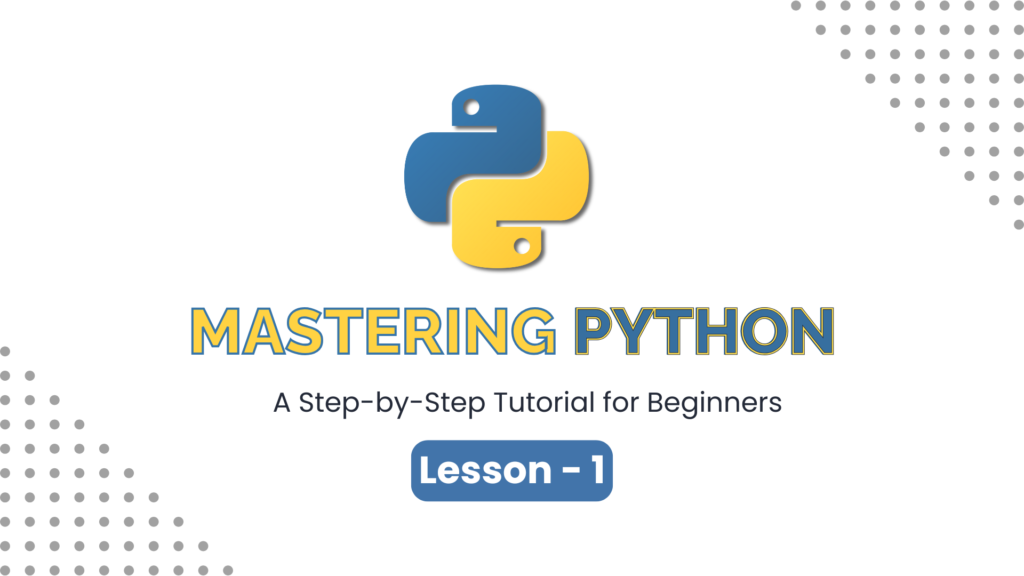
What is Python?
Python is a high-level, interpreted programming language designed for simplicity and readability. Developed by Guido van Rossum and released in 1991, it has since grown to become one of the most popular languages in the world due to its versatility and ease of use. Python’s syntax is clear and concise, making it an excellent choice for beginners, while its robust libraries make it powerful enough for experts.
History and Features of Python
- History: Python was created in the late 1980s and its first release was in 1991. It was conceived as a successor to the ABC language, focusing on simplicity and ease of use. Over the years, Python has grown with multiple versions, the most current being Python 3.x.
- Key Features:
- Interpreted: Python executes code line by line, which makes debugging easier.
- High-Level Language: Python abstracts away many of the complexities of lower-level programming languages, enabling developers to focus on the core logic.
- Dynamically Typed: Variables in Python don’t need explicit declaration of their type.
- Cross-Platform: Python runs on many platforms, including Windows, Mac, Linux, and more.
- Extensive Libraries: Python has a rich ecosystem of libraries for various tasks such as web development, data manipulation, machine learning, and automation.
Why Python is Popular?
Python’s popularity can be attributed to several reasons:
- Readability: Python’s simple, English-like syntax makes it easy to read and write code.
- Simplicity: Python’s clear structure reduces the cognitive load for developers, making it great for beginners.
- Versatility: From web development to data science, Python can be used for a wide range of applications.
- Large Community and Ecosystem: Python has a massive community and numerous frameworks and libraries that speed up development.
- Portability: Python code runs on multiple platforms without requiring modification.
Python Use Cases
Python’s versatility makes it ideal for a variety of applications:
- Web Development: Frameworks like Django and Flask enable the creation of robust, scalable web applications.
- Data Science: Libraries like NumPy, Pandas, and Matplotlib make Python a popular choice for data manipulation and analysis.
- Machine Learning: Python offers powerful tools for machine learning through libraries like TensorFlow, Scikit-learn, and Keras.
- Automation/Scripting: Python can automate repetitive tasks, from simple file manipulations to complex system processes.
- Scripting for DevOps: Python’s flexibility allows it to be used in automating deployment pipelines and managing infrastructure.
Installing Python
Here’s how to install Python on different operating systems:
- Windows:
- Go to the official Python website.
- Download the latest Python 3.x version for Windows.
- Run the installer, ensuring you check the box “Add Python to PATH”.
- Once installed, verify the installation by opening Command Prompt and typing:
python --version
Mac:
- macOS comes with Python 2.x pre-installed. To install the latest Python 3.x, download it from the Python website.
- Run the installer and follow the instructions.
- Verify the installation using:
python3 --version
Linux:
- Most Linux distributions come with Python pre-installed. You can check the installed version by running:
python3 --version
If Python is not installed, use your package manager to install it:
sudo apt-get install python3
Setting Up Your Python Environment
- Choosing an IDE: Python code can be written in any text editor, but it’s recommended to use an IDE or text editor designed for development. Here are some popular options:
- VSCode: A lightweight, yet powerful editor with Python extensions available.
- PyCharm: A dedicated Python IDE with features like code completion, debugging, and testing.
- Jupyter Notebook: Often used in data science, Jupyter allows you to write and execute code in a cell-based format.
- Installing an IDE (Example: VSCode):
- Download and install VSCode from the official site.
- Once installed, open VSCode and search for the Python extension in the marketplace.
- Install the extension to enable Python-specific features, such as code linting, syntax highlighting, and debugging.
- Verifying Installation:
- To ensure Python and your IDE are correctly set up, create a simple “Hello World” program:
print("Hello, Python World!")
-
- Run the program in your IDE or terminal to see the output.
By the end of this lesson, you’ll have a basic understanding of Python, its popularity, installation process, and how to set up a Python development environment. In the next lesson, we’ll dive deeper into Python basics like variables, data types, and control structures.