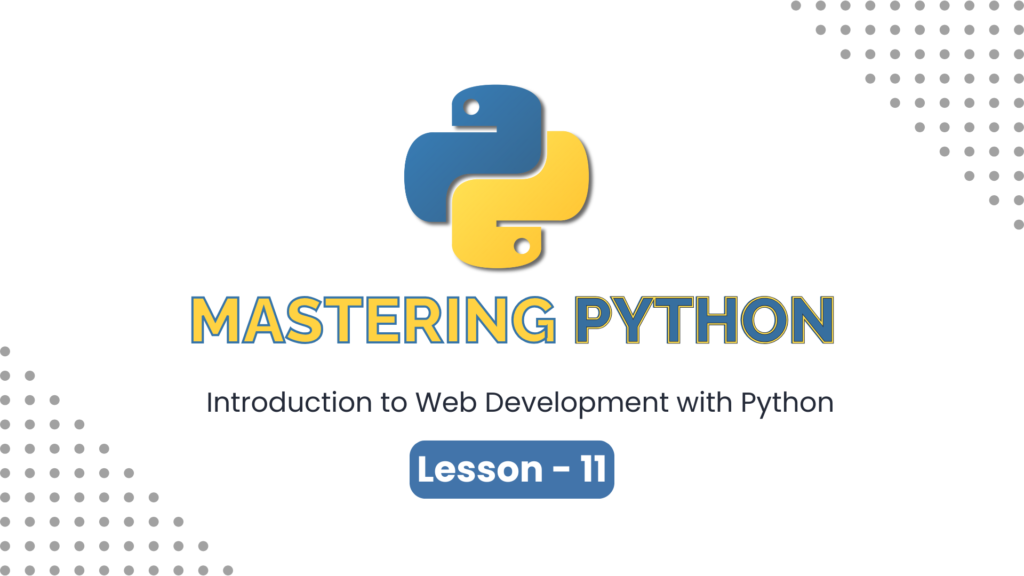
Python’s versatility extends to web development, offering powerful frameworks that make it easier to build dynamic, scalable, and feature-rich web applications. This lesson introduces the basics of web development with Python, focusing on the Flask framework.
1. Python Frameworks for Web Development
Python provides several frameworks to simplify web development. The two most popular are:
- Flask: A lightweight and flexible micro-framework. It is easy to learn and ideal for small to medium-scale applications.
- Django: A high-level framework that follows the “batteries-included” philosophy. It is great for building complex, scalable applications.
2. Setting Up Flask
To start with Flask, follow these steps:
- Install Flask:
pip install flask
2. Create a Flask Application:
Save the following code in a file named app.py
:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Welcome to Flask Web Development!"
if __name__ == '__main__':
app.run(debug=True)
3. Run the Application:
Open your terminal, navigate to the directory containing app.py
, and run:
python app.py
- Open
http://127.0.0.1:5000/
in your browser to see your application running.
3. Handling Routes and Requests
In Flask, routes define the URLs your application responds to. Use the @app.route
decorator to map URLs to Python functions.
Example of handling routes and requests:
from flask import Flask, request
app = Flask(__name__)
@app.route('/')
def home():
return "Welcome to the Home Page!"
@app.route('/greet', methods=['GET'])
def greet():
name = request.args.get('name', 'Guest')
return f"Hello, {name}!"
if __name__ == '__main__':
app.run(debug=True)
Use the request
object to handle query parameters and form data.
4. Rendering HTML Templates
Flask uses Jinja2, a powerful templating engine, to render dynamic HTML pages.
- Create a Template: Save the following code as
templates/home.html
:
<!DOCTYPE html>
<html>
<head>
<title>Flask Web App</title>
</head>
<body>
<h1>Welcome to {{ site_name }}!</h1>
</body>
</html>
2. Render the Template in Flask:
Update your Flask application to include template rendering:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html', site_name="My Flask App")
if __name__ == '__main__':
app.run(debug=True)
When you visit http://127.0.0.1:5000/
, the HTML page with dynamic content will be displayed.
Summary
This lesson introduced the basics of web development with Python using Flask:
- Explored Python frameworks like Flask and Django.
- Set up a basic Flask application.
- Defined routes and handled requests.
- Rendered dynamic HTML templates with Jinja2.
In the next lesson, we’ll delve deeper into advanced Flask features and explore Django to understand how these frameworks empower modern web development.