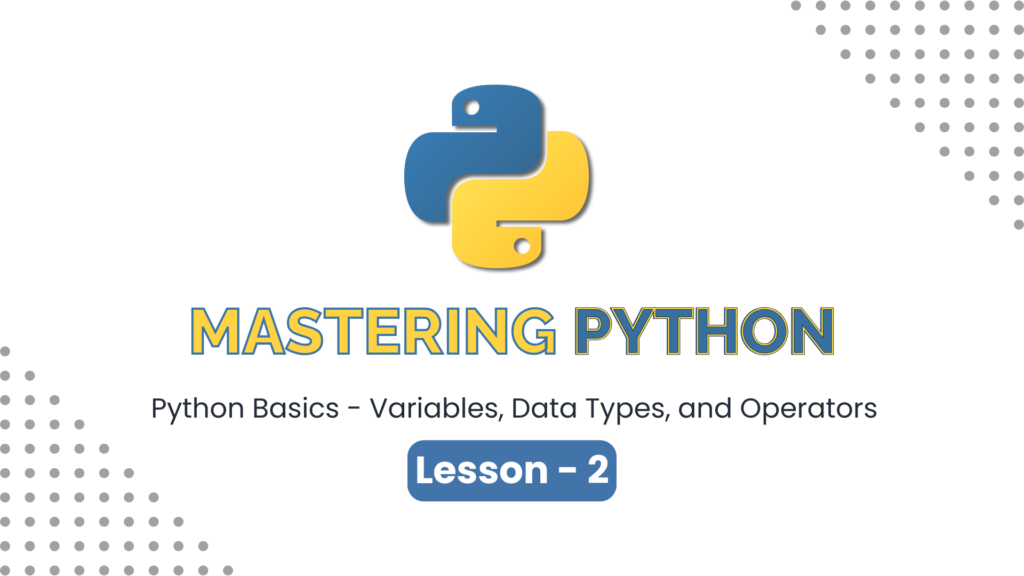
In this lesson, we’ll explore the building blocks of Python: variables, data types, and operators. These are fundamental concepts that will form the foundation of your Python programming knowledge.
1. Variables in Python
A variable in Python is a name assigned to a value. It acts as a container for storing data that can be used and manipulated throughout the program.
Syntax:
variable_name = value
- Python does not require explicit declaration of variable types, making it a dynamically typed language.
Example:
x = 10
name = "Alice"
is_valid = True
In this example, x
is an integer, name
is a string, and is_valid
is a boolean.
2. Data Types in Python
Python supports several built-in data types, and understanding them is crucial to effectively working with variables.
Here are the most common data types in Python:
- Integers: Whole numbers, e.g.,
10
,-5
,0
.
age = 25
Floats: Numbers with decimal points, e.g., 3.14
, 0.99
.
pi = 3.14
Strings: A sequence of characters enclosed in quotes, e.g., "Hello, World!"
.
greeting = "Hello, Python!"
Booleans: Represent True or False values.
is_active = True
Lists: Ordered, mutable collections of items.
colors = ["red", "green", "blue"]
Tuples: Ordered, immutable collections of items.
point = (5, 10)
Dictionaries: Key-value pairs, where each key is unique.
person = {"name": "Alice", "age": 25}
Python automatically detects the data type based on the value assigned, but you can also use the type()
function to check the data type.
Example:
x = 10
print(type(x)) # Output: <class 'int'>
3. Operators in Python
Operators allow you to perform operations on variables and values. Python supports several types of operators:
a) Arithmetic Operators
Used for basic mathematical operations.
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus (remainder)**
: Exponentiation//
: Floor Division (rounds down)
Example:
a = 10
b = 3
print(a + b) # Output: 13
print(a / b) # Output: 3.3333333333333335
print(a // b) # Output: 3
b) Comparison Operators
Used to compare two values, returning True
or False
.
==
: Equal to!=
: Not equal to>
: Greater than<
: Less than>=
: Greater than or equal to<=
: Less than or equal to
Example:
x = 5
y = 10
print(x > y) # Output: False
print(x == 5) # Output: True
c) Logical Operators
Used to combine conditional statements.
and
: ReturnsTrue
if both statements are true.or
: ReturnsTrue
if one of the statements is true.not
: Inverts the boolean value.
Example:
x = 5
y = 10
print(x > 2 and y < 20) # Output: True
print(not x == 5) # Output: False
d) Assignment Operators
Used to assign values to variables.
=
: Assigns value.+=
: Adds and assigns.-=
: Subtracts and assigns.*=
: Multiplies and assigns./=
: Divides and assigns.
Example:
x = 5
x += 3 # Same as x = x + 3
print(x) # Output: 8
4. Working with Input and Output
You can get user input using the input()
function and display output using the print()
function.
Example:
name = input("Enter your name: ")
print("Hello, " + name + "!")
Here, input()
allows the user to enter a value, which is stored in the variable name
. The print()
function then outputs a greeting.
5. Comments in Python
Comments are lines in the code that are not executed. They are used to provide explanations or notes for developers. You can write comments using the #
symbol.
Example:
# This is a comment
x = 10 # Assigning value to x
Conclusion
In this lesson, you’ve learned the basics of Python, including variables, data types, and operators. These foundational concepts are essential as you progress in your Python journey. Next, we’ll dive deeper into control structures like loops and conditionals, which are critical for writing more complex programs.