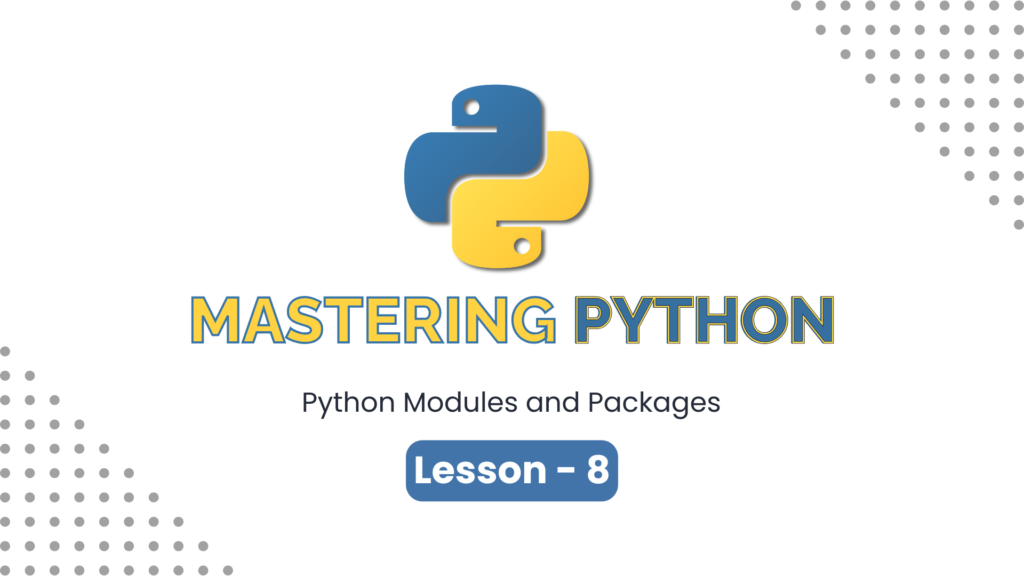
Python modules and packages allow you to organize and reuse your code efficiently, making your programs modular and scalable. In this lesson, we’ll explore how to work with modules and packages in Python.
1. What Are Modules in Python?
A module is a single Python file containing definitions and statements. You can reuse these across multiple programs.
Examples:
- Built-in modules like
math
,os
,random
. - Custom modules created by developers.
Using a Module:
import math
print(math.sqrt(16)) # Output: 4.0
2. What Are Packages in Python?
A package is a directory containing multiple modules and a special __init__.py
file that marks it as a package.
Structure Example:
mypackage/
__init__.py
module1.py
module2.py
Using a Package:
from mypackage import module1
module1.function_name()
3. Creating and Using Custom Modules
Step 1: Create a .py
file (e.g., my_module.py
).
Step 2: Add functions or variables to the file.
# my_module.py
def greet(name):
return f"Hello, {name}!"
Step 3: Import and use it in your program.
import my_module
print(my_module.greet("Alice")) # Output: Hello, Alice!
4. Creating and Using Custom Packages
Step 1: Create a directory with an __init__.py
file.
Step 2: Add modules to the directory.
Example Structure:
math_tools/
__init__.py
operations.py
constants.py
Step 3: Use the package in your project.
from math_tools.operations import add
print(add(5, 10)) # Output: 15
5. Importing Modules and Packages
- Standard Import:
import module_name
- Alias Import:
import module_name as alias
- Specific Import:
from module_name import function_name
- Wildcard Import:
from module_name import *
6. Python Standard Library
Python’s standard library includes powerful modules for common tasks.
os
: For file and directory operations.sys
: For system-specific parameters and functions.random
: For random number generation.datetime
: For date and time manipulation.
Example:
import random
print(random.randint(1, 100)) # Output: Random number between 1 and 100
7. Installing and Using External Packages
Python’s rich ecosystem includes thousands of external packages hosted on PyPI (Python Package Index). You can install them using pip
.
Installing a Package:
pip install requests
Using the Package:
import requests
response = requests.get("https://api.example.com")
print(response.status_code)
Key Takeaways
- Modules group related functions and variables into reusable files.
- Packages allow you to organize your modules for better maintainability.
- Python’s standard library and external packages offer endless possibilities for development.