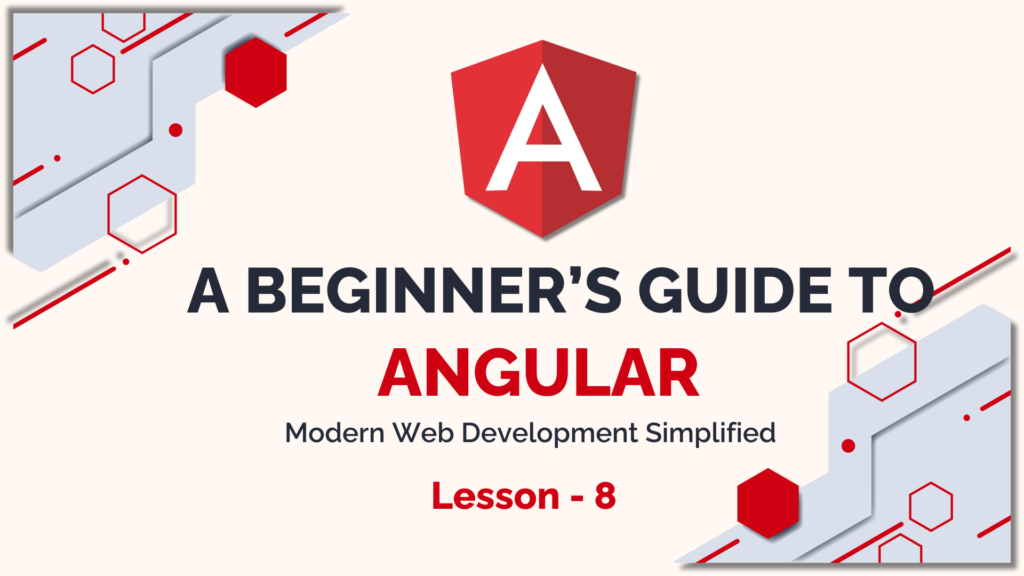
we will explore Pipes in Angular. Pipes are a powerful feature that allow you to transform data directly in your templates. Whether you need to format dates, currencies, or manipulate text, Angular pipes can help streamline your data presentation with minimal effort.
1. Introduction to Pipes in Angular
Pipes are a mechanism for transforming or formatting data in Angular templates. They allow you to display data in a desired format without altering the original data. Pipes can be used in interpolation, property binding, and directives.
2. Built-in Pipes in Angular
Angular provides a set of built-in pipes that are ready to use for common data transformations. Below are some of the most commonly used built-in pipes:
- 1. DatePipe: Formats a date value according to locale rules.
{{ today | date }} <!-- Output: Oct 8, 2024 -->
{{ today | date: 'short' }} <!-- Output: 10/8/24, 9:00 AM -->
2. UpperCasePipe & LowerCasePipe: Transforms text to uppercase or lowercase.
{{ 'hello world' | uppercase }} <!-- Output: HELLO WORLD -->
{{ 'HELLO WORLD' | lowercase }} <!-- Output: hello world -->
3. CurrencyPipe: Formats a number as currency.
{{ price | currency }} <!-- Output: $123.45 -->
{{ price | currency: 'EUR' }} <!-- Output: €123.45 -->
4. DecimalPipe: Transforms a number into a string with a specified number of digits.
{{ pi | number: '1.2-2' }} <!-- Output: 3.14 -->
5. PercentPipe: Transforms a number into a percentage.
{{ 0.25 | percent }} <!-- Output: 25% -->
6. JsonPipe: Converts a JavaScript object into a JSON string.
{{ myObject | json }}
7. SlicePipe: Returns a subset of an array or string.
{{ myString | slice:0:5 }} <!-- Output: Hello -->
8. KeyValuePipe: Transforms an object or map into an array of key-value pairs.
<div *ngFor="let item of myObject | keyvalue">
{{ item.key }}: {{ item.value }}
</div>
3. Using Pipes in Angular Templates
Using pipes is straightforward. Simply use the pipe (|
) operator to apply a pipe in the template:
{{ expression | pipeName }}
You can also chain multiple pipes together:
{{ price | currency | uppercase }} <!-- Output: $123.45 in uppercase -->
4. Parameterizing Pipes
Some pipes, like the DatePipe
and CurrencyPipe
, accept additional arguments. You can pass parameters to the pipe after a colon (:
):
{{ today | date: 'fullDate' }} <!-- Output: Tuesday, October 8, 2024 -->
{{ price | currency: 'INR' }} <!-- Output: ₹123.45 -->
5. Creating Custom Pipes
While Angular provides a variety of built-in pipes, you may need to create custom pipes to meet specific needs. Here’s how you can create a custom pipe:
- 1. Generate a Pipe using Angular CLI:
ng generate pipe custom
2. Implement the Pipe Logic in the generated pipe file:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'customPipe'
})
export class CustomPipe implements PipeTransform {
transform(value: string, ...args: any[]): string {
// Custom transformation logic here
return value.split('').reverse().join(''); // Example: reverse a string
}
}
3. Use the Custom Pipe in your template:
{{ 'Angular' | customPipe }} <!-- Output: ralugnA -->
6. Pure and Impure Pipes
Pipes in Angular can be pure or impure:
- Pure Pipes: These pipes are executed only when the input value changes. Angular pipes are pure by default, which makes them efficient and prevents unnecessary recalculations.
- Impure Pipes: These pipes are recalculated on every change detection cycle, regardless of whether the input has changed or not. Use impure pipes cautiously to avoid performance issues.
To mark a pipe as impure, set pure: false
in the @Pipe
decorator:
@Pipe({
name: 'impurePipe',
pure: false
})
7. Performance Considerations
Pipes help reduce the need for complex logic in the component, but there are some performance considerations:
- Avoid using impure pipes unless absolutely necessary, as they are re-evaluated frequently.
- For large datasets, consider using pagination or infinite scroll to limit the amount of data processed by pipes like
SlicePipe
. - Always use pure pipes to ensure optimal performance.
8. Conclusion
Pipes are a powerful feature in Angular that help you transform data effortlessly in your templates. Whether you are formatting dates, numbers, or creating custom transformations, pipes make it easy to present your data in the desired format. Understanding how to effectively use both built-in and custom pipes is essential for building clean and efficient Angular applications.